Back
Project 5
Turn off the light freely!
- Project Description -
Lazy People —— A remote control device for controlling finger shaking
Inspiration source : In life, the phenomenon of forgetting to turn off the light occurs from time to time, such as out of the toilet only to find the light is not off, and then we usually do not bother to run again, so we developed a lazy person to turn off the light artifact, you can freely control the light switch on the phone.
Organization logic : Connect aliyun server with wifi module, assign "1" to be on and "0" to be off. After scanning the code, the phone can be controlled through the button on the phone.
- Materials -
-
1x Arduino Uno
-
1 x WiFi module
-
2 x steering gear
- Fritzing -
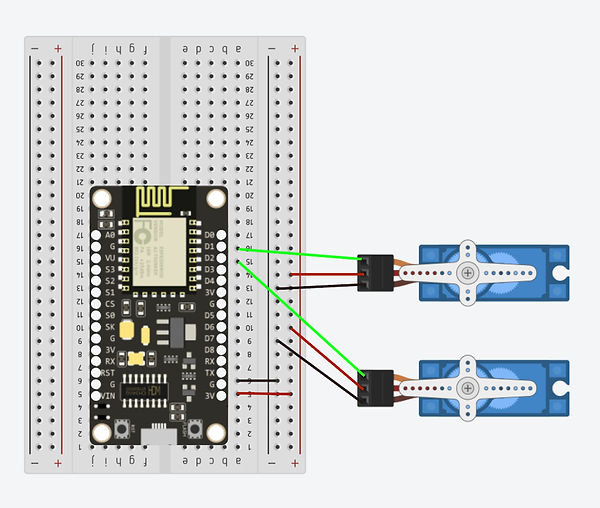
- Code -
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
#include <ArduinoJson.h>
#include <aliyun_mqtt.h>
#include <Servo.h> //servo库
Servo servo1; //创建舵机对象来控制舵机
Servo servo2; //创建舵机对象来控制舵机
#define SENSOR_PIN 13 //pin define
#define LED D4
//#define LED2 D5
#define PRODUCT_KEY "a1VgxFuS6Po"//exchange PRODUCT_KEY
#define DEVICE_NAME "led1"//exchange DEVICE_NAME
#define DEVICE_SECRET "a7305306fcb944620dcb17447a5066ea"//exchange DEVICE_SECRET
#define DEV_VERSION "S-TH-WIFI-v1.0-20190220"
#define WIFI_SSID "五号江景房"//exchange WIFI
#define WIFI_PASSWD "805JJF.."//exchange WIFI password
#define ALINK_BODY_FORMAT "{\"id\":\"123\",\"version\":\"1.0\",\"method\":\"%s\",\"params\":%s}"
#define ALINK_TOPIC_PROP_POST "/sys/" PRODUCT_KEY "/" DEVICE_NAME "/thing/event/property/post"
#define ALINK_TOPIC_PROP_POSTRSP "/sys/" PRODUCT_KEY "/" DEVICE_NAME "/thing/event/property/post_reply"
#define ALINK_TOPIC_PROP_SET "/sys/" PRODUCT_KEY "/" DEVICE_NAME "/thing/service/property/set"
#define ALINK_METHOD_PROP_POST "thing.event.property.post"
#define ALINK_TOPIC_DEV_INFO "/ota/device/inform/" PRODUCT_KEY "/" DEVICE_NAME ""
#define ALINK_VERSION_FROMA "{\"id\": 123,\"params\": {\"version\": \"%s\"}}"
unsigned long lastMs = 0;
int val=0;
int servo1Pin = D2;
int servo2Pin = D3;
WiFiClient espClient;
PubSubClient mqttClient(espClient);
void init_wifi(const char *ssid, const char *password)
{
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED)
{
Serial.println("WiFi does not connect, try again ...");
delay(500);
}
Serial.println("Wifi is connected.");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
}
void mqtt_callback(char *topic, byte *payload, unsigned int length)
{
Serial.print("Message arrived [");
Serial.print(topic);
Serial.print("] ");
payload[length] = '\0';
Serial.println((char *)payload);
Serial.println("");
Serial.println((char *)payload);
Serial.println("");
if (strstr(topic, ALINK_TOPIC_PROP_SET))
{
StaticJsonBuffer<500> jsonBuffer;
JsonObject &root = jsonBuffer.parseObject(payload);
int params_LightSwitch = root["params"]["LightSwitch"]; //终端命令获取
int params_FanSwitch = root["params"]["FanSwitch"]; //终端命令获取
Serial.println(params_LightSwitch);
Serial.println(params_FanSwitch);
if(params_LightSwitch == 1 && servo1.read() == 0)
{
Serial.println("LED ON");
servo1.attach(servo1Pin);
delay(1);
//servo.write(0);
delay(1000);
servo1.write(70);
delay(2000);
//servo1.detach();
}
else{
Serial.println("LED OFF");
servo1.attach(servo1Pin);
delay(1);
//servo.write(0);
delay(1000);
servo1.write(0);
delay(2000);
//servo1.detach();
}
if(params_FanSwitch == 1 && servo2.read() == 0)
{
Serial.println("FAN ON");
servo2.attach(servo2Pin);
delay(1);
//servo.write(0);
delay(1000);
servo2.write(70);
delay(2000);
//servo2.detach();
}
else
{
Serial.println("FAN OFF");
servo2.attach(servo2Pin);
delay(1);
//servo.write(0);
delay(1000);
servo2.write(0);
delay(2000);
//servo2.detach();
}
if (!root.success())
{
Serial.println("parseObject() failed");
return;
}
}
}
void mqtt_version_post()
{
char param[512];
char jsonBuf[1024];
//sprintf(param, "{\"MotionAlarmState\":%d}", digitalRead(13));
sprintf(param, "{\"id\": 123,\"params\": {\"version\": \"%s\"}}", DEV_VERSION);
// sprintf(jsonBuf, ALINK_BODY_FORMAT, ALINK_METHOD_PROP_POST, param);
Serial.println(param);
mqttClient.publish(ALINK_TOPIC_DEV_INFO, param);
}
void mqtt_check_connect()
{
while (!mqttClient.connected())//
{
while (connect_aliyun_mqtt(mqttClient, PRODUCT_KEY, DEVICE_NAME, DEVICE_SECRET))
{
Serial.println("MQTT connect succeed!");
//client.subscribe(ALINK_TOPIC_PROP_POSTRSP);
mqttClient.subscribe(ALINK_TOPIC_PROP_SET);
Serial.println("subscribe done");
mqtt_version_post();
}
}
}
void mqtt_interval_post()
{
char param[512];
char jsonBuf[1024];
int servoNum1 = servo1.read();
int servoNum2 = servo2.read();
int state1 = 1;
int state2 = 1;
if(servoNum1 == 0)
{
state1 = 0;
}
if(servoNum2 == 0)
{
state2 = 0;
}
//sprintf(param, "{\"MotionAlarmState\":%d}", digitalRead(13));
//sprintf(param, "{\"LightSwitch\":%d,\"range\":%d}",!digitalRead(LED),val);
sprintf(param, "{\"LightSwitch\":%d,\"FanSwitch\":%d}",state1,state2); //设备上报
sprintf(jsonBuf, ALINK_BODY_FORMAT, ALINK_METHOD_PROP_POST, param);
Serial.println(jsonBuf);
mqttClient.publish(ALINK_TOPIC_PROP_POST, jsonBuf);
}
void setup()
{
pinMode(SENSOR_PIN, INPUT);
pinMode(LED, OUTPUT);
//pinMode(LED2, OUTPUT);
servo1.attach(servo1Pin);//把连接在引脚D1上的舵机赋予舵机对其控制
servo1.write(0); //close cap on power on
delay(500);
servo1.detach();
servo2.attach(servo2Pin);//把连接在引脚D2上的舵机赋予舵机对其控制
servo2.write(0); //close cap on power on
delay(500);
servo2.detach();
/* initialize serial for debugging */
Serial.begin(115200);
Serial.println("Demo Start");
init_wifi(WIFI_SSID, WIFI_PASSWD);
mqttClient.setCallback(mqtt_callback);
}
// the loop function runs over and over again forever
void loop()
{
/*val= analogRead(A0);
if (val>500)
{digitalWrite(LED2,HIGH);}
else
{digitalWrite(LED2,LOW);}*/
if (millis() - lastMs >= 5000)
{
lastMs = millis();
mqtt_check_connect();
/* Post */
mqtt_interval_post();
}
mqttClient.loop();
/*unsigned int WAIT_MS = 2000;
if (digitalRead(SENSOR_PIN) == HIGH)
{
Serial.println("Motion detected!");
}
else
{
Serial.println("Motion absent!");
}
delay(WAIT_MS); // ms
Serial.println(millis() / WAIT_MS);*/
}
Project Process
—— processing——
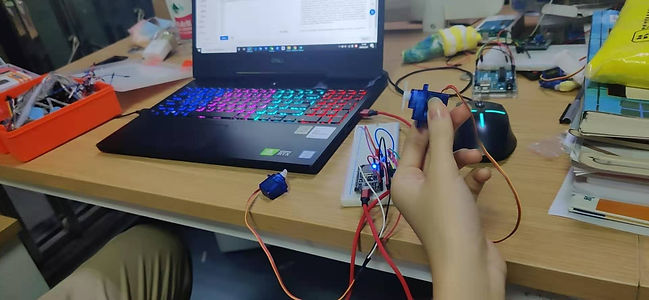
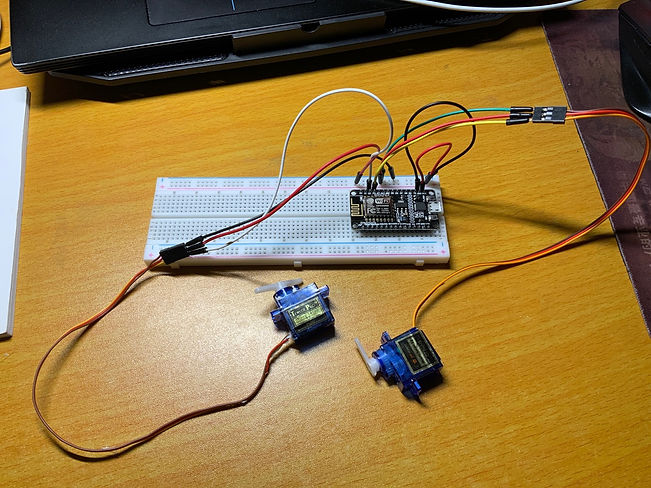
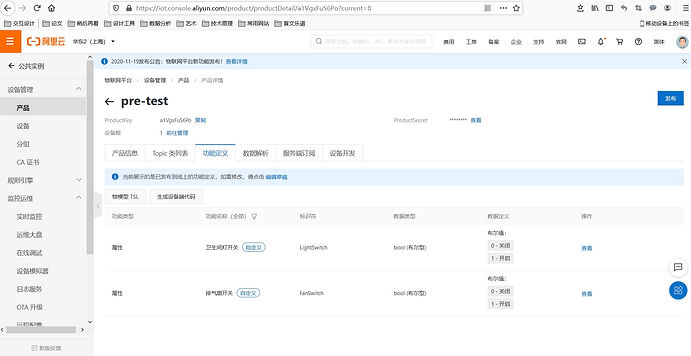
Deploy on aliyun
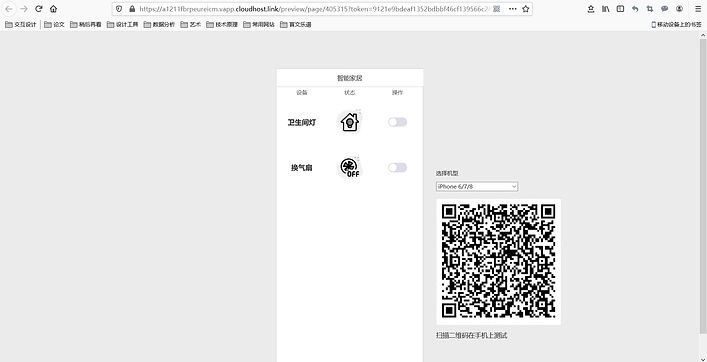
Edit the mobile interface and generate the QR code, which can be controlled by the mobile phone after scanning the code
—— final effect ——

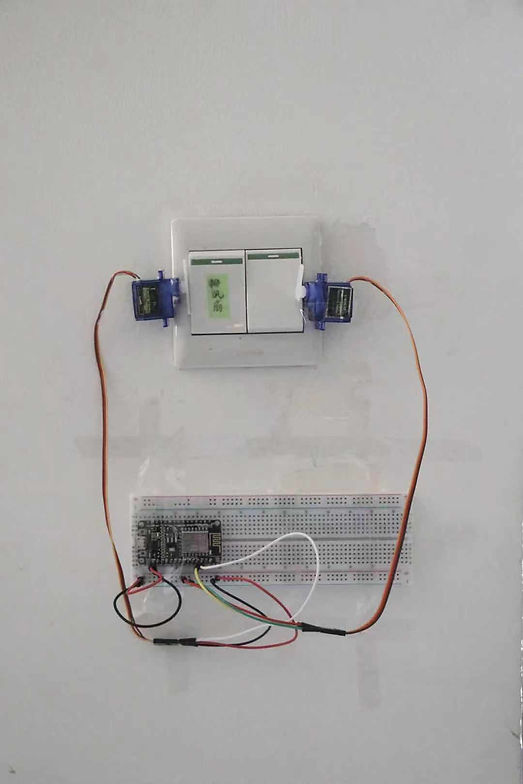
—— problem ——
1. The steering gear cannot be fixed, and the blades will be offset during rotation.
2. Aliyun data transmission is unstable and the default value is 0, so the exhaust fan will automatically turn off after the toilet light is turned on.
—— solution ——
For now, fix it by hand, and then look for other materials that can be fixed.